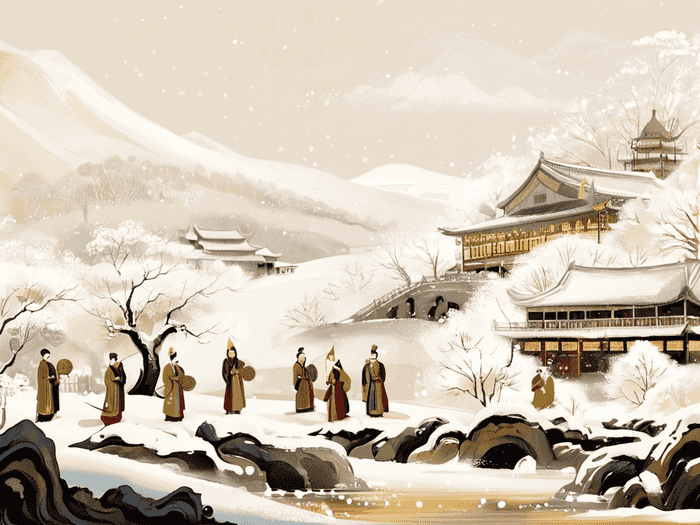
风雨同舟为您分享以下优质知识
判断二进制数中1的个数是计算机科学中的常见问题,可以通过多种方法实现。以下是几种常用且高效的方法:
一、位运算高效方法
通过不断消除最右边的1来统计1的个数,时间复杂度为O(k),其中k是1的个数。
```c
int countones(int n) {
int count = 0;
while (n) {
n &= (n - 1);
count++;
}
return count;
}
```
`n & -n` 位翻转法
利用补码特性,`n & -n` 可以快速翻转所有1位,时间复杂度为O(1)。
```c
int countones(int n) {
int count = 0;
while (n) {
n = n & -n;
count++;
}
return count;
}
```
二、分治法(按位段统计)
将32位整数分成4段,分别统计每段中1的个数,再相加。此方法时间复杂度为O(1)。
```c
int countones(int n) {
int count = 0;
for (int i = 0; i < 4; i++) {
int mask = 0x55555555 >
i;
}
return count;
}
```
三、查表法(预计算)
对于固定范围的整数,可以预先计算每个数的1的个数,存储在数组中,查询时直接查表。
四、其他方法
简单除暴法:
逐位检查,时间复杂度为O(32)=O(1)。
```c
int countones(int n) {
int count = 0;
while (n) {
count += n & 1;
n >
>
= 1;
}
return count;
}
```
使用`bin()`函数转换为二进制字符串,统计'1'的个数。
```python
def count_ones(n):
return bin(n)[2:].count('1')
```
注意事项
负数处理:上述方法对负数有效,因为C语言中`int`是32位有符号整数,`n & (n - 1)`会正确处理补码表示。
性能对比:`n & (n - 1)`和`n & -n`性能最佳,适用于大多数场景;分治法适用于需要扩展到更大整数的场景。
通过以上方法,可以高效地统计二进制数中1的个数。