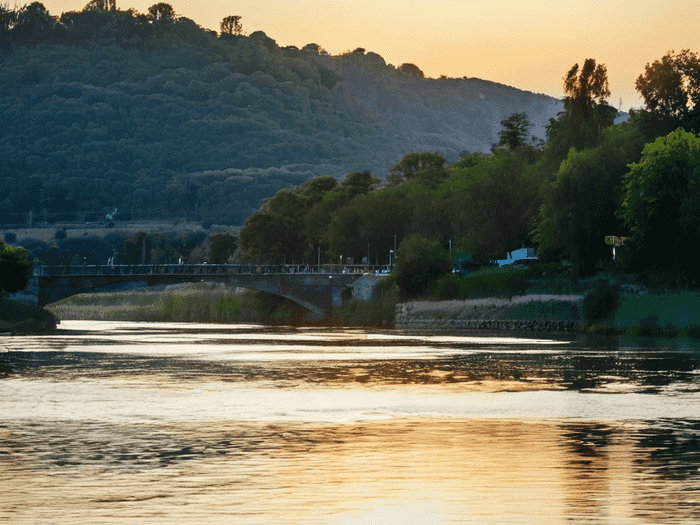
心有灵犀为您分享以下优质知识
将二进制数据转换为数组的方法因编程语言和数据格式不同而有所差异,以下是主要方法及示例:
一、Python实现
使用`struct`模块解析二进制文件,需先确定数据格式(如整数、浮点数等)。例如,将3个整数的二进制数据转换为二维数组:
```python
import struct
binary_data = b'x01x00x00x00x02x00x00x00x03x00x00x00'
data_format = 'iiii'
data = struct.unpack(data_format, binary_data)
array_2d = [data[i:i+2] for i in range(0, len(data), 2)]
print(array_2d) 输出: [[1, 2], [3, 4]]
```
二进制字符串转数组
使用`numpy`或列表推导式快速转换。例如:
```python
import numpy as np
bin_string = "1011"
array = np.array([int(c) for c in bin_string])
print(array) 输出: [1 0 1 1]
```
二、C语言实现
文件转字符数组
使用`fread`读取二进制文件并存储到字符数组中:
```c
include
include
int main() {
FILE *file = fopen("test.bin", "rb");
unsigned char *buf = malloc(100 * sizeof(unsigned char));
fread(buf, 1, 100, file);
fclose(file);
// buf 现在包含文件内容
return 0;
}
```
二进制数据转结构化数组
需定义数据结构并使用`memcpy`或`struct`解析:
```c
include
include
struct Data {
int a;
int b;
int c;
};
int main() {
struct Data data;
fread(&data, sizeof(struct Data), 1, stdin);
// data 现在包含解析后的数据
return 0;
}
```
三、其他工具与方法
十六进制转数组:
通过文本处理工具(如Excel)将十六进制字符串转换为字节数组,或使用Python脚本批量处理。
数据库中的转换:在Flink等流处理框架中,使用`from_base64`或自定义标量函数将二进制数据转换为数组。
注意事项:转换前需明确数据格式(如位数、类型、顺序),避免解析错误。处理大文件时建议使用缓冲区或流式读取。