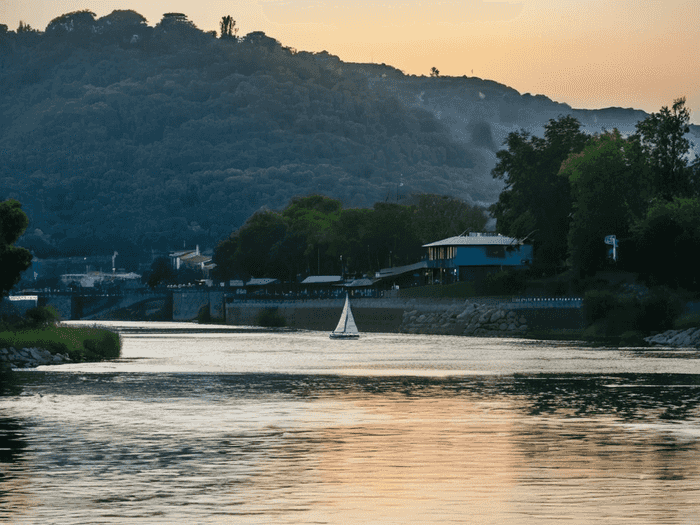
抓住机遇为您分享以下优质知识
在Java中判断文件是否为二进制文件,可以通过以下两种常用方法实现:
一、通过字符编码检测
通过`InputStreamReader`以字符模式读取文件,尝试使用常见字符集(如UTF-8)解码。如果解码失败(抛出`UnsupportedEncodingException`)或解码后出现乱码,则可判断为二进制文件。
```java
import java.io.*;
public static boolean isBinaryFile(String fileName) {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(fileName), "UTF-8"))) {
String line = reader.readLine();
return line != null; // 若能正常读取一行,则可能是文本文件
} catch (UnsupportedEncodingException e) {
return true; // 字符集不支持,判定为二进制
} catch (IOException e) {
return true; // 读取失败,可能是二进制文件
}
}
```
逐字符检测
读取文件中的每个字符,检查是否全为可打印字符(如ASCII字符)。若存在非打印字符(如0x00-0x1F或0x7F-0x9F),则判定为二进制文件。
```java
import java.io.*;
public static boolean isBinaryFile(String fileName) {
try (FileInputStream fis = new FileInputStream(fileName)) {
int data;
while ((data = fis.read()) != -1) {
if (!isPrintableChar(data)) {
return true; // 存在非打印字符
}
}
} catch (IOException e) {
return true; // 读取失败,可能是二进制文件
}
return false; // 全部为可打印字符
}
private static boolean isPrintableChar(int ch) {
return (ch >
= 32 && ch 0) {
int data = fis.read();
if (isPrintableChar(data)) {
printableCount++;
}
totalBytes -= 16; // 每次读取16字节
}
return (double) printableCount / totalBytes < 0.2; // 可打印字符占比低于20%
} catch (IOException e) {
return true; // 读取失败,可能是二进制文件
}
}
```
使用位操作检测
通过位操作检查文件中是否存在连续的0或1块。若存在较长的连续0或1序列,则可能是二进制文件。
```java
import java.io.*;
public static boolean isBinaryFile(String fileName) {
try (FileInputStream fis = new FileInputStream(fileName)) {
byte[] buffer = new byte;
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
for (int i = 0; i < bytesRead; i++) {
if ((buffer[i] & 0x0F) == 0x0F) { // 检测连续4个0
return true;
} else if ((buffer[i] & 0xF0) == 0xF0) { // 检测连续4个1
return true;
}
}
}
} catch (IOException e) {
return true; // 读取失败,可能是二进制文件
}
return false; // 未检测到连续0/1块
}
```
注意事项
编码问题:
所有文件本质上是二进制,文本文件只是特定编码的字符集合。若需处理文本文件,建议显式指定字符集(如`UTF-8`)。
性能优化:对于大文件,逐字符或逐块读取并检测效率较低。可根据需求选择合适的方法,例如统计字符频率或使用位操作优化检测逻辑。
通过上述方法,可根据具体场景