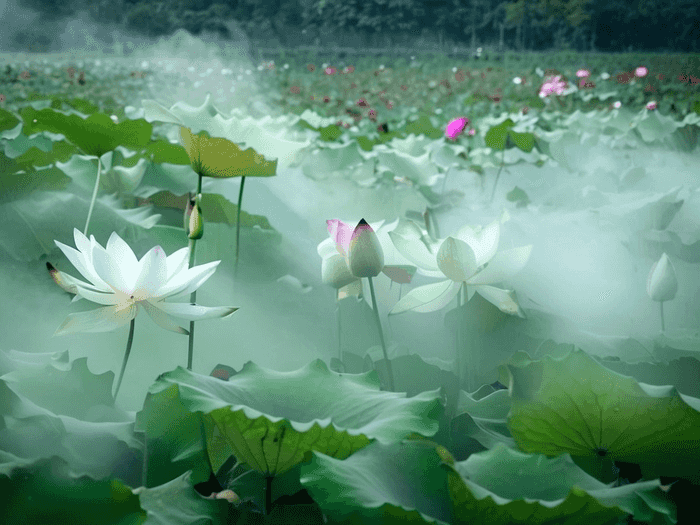
诚信公仆为您分享以下优质知识
将IP地址转换为二进制地址的方法主要有以下两种,结合权威性和实用性推荐使用:
一、手动计算法(适用于理解原理)
将IP地址按点号分割为四段(如192.168.1.1),每段转换为8位二进制。
二进制转换
- 除2取余法:
将每段十进制数连续除以2,记录余数(1或0),从低位到高位排列。例如192÷2=96...0,96÷2=48...0,依次类推得到11000000。
- 减法法:用每段数依次减去128、64、32、16、8、4、2、1,记录能减的次数(1表示该位为1),剩余为0则该位为0。例如192-128=64,记录1;64-64=0,记录1,最终得到11000000。
二、编程实现法(高效便捷)
使用编程语言(如C++)实现自动化转换,核心步骤如下:
将IP地址按点号分割为四段字符串。
逐段转换
对每段字符串转换为整数,再通过位运算或循环除2取余得到二进制表示。
组合输出
将四段二进制字符串用点号连接,形成标准32位二进制IP。
示例代码(C++):
```cpp
include
include
include
include
include
std::string bin_to_dec(const std::string& s) {
std::stack st;
std::stringstream ss(s);
std::string token;
while (std::getline(ss, token, '.')) {
st.push(std::stoi(token));
}
std::string binary(32, '0');
for (int i = 0; i < 32; ++i) {
binary[i] = st.top() % 2 + '0';
st.pop();
}
return binary;
}
int main() {
std::string ip = "192.168.1.1";
std::string binary_ip = bin_to_dec(ip);
std::cout