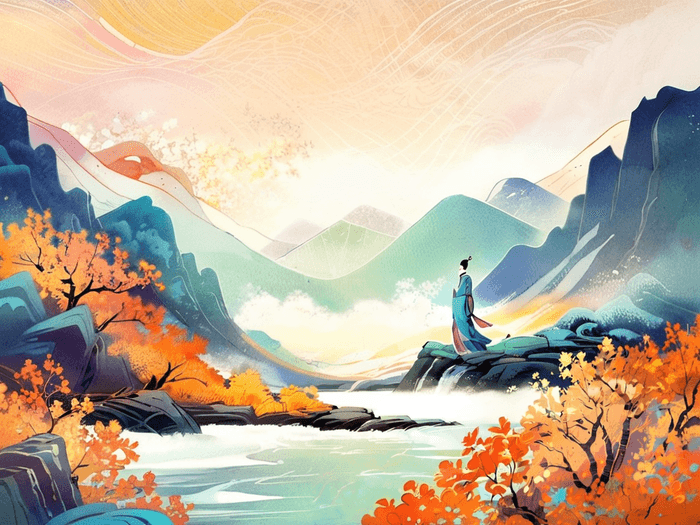
心有灵犀为您分享以下优质知识
以下是使用Python生成随机学号和成绩的完整方法,结合了学号生成、成绩生成及数据处理的完整流程:
一、生成随机学号
学号通常由固定长度的数字组成,例如6位整数。可以使用`random.randint`函数生成指定范围的随机整数。
```python
import random
def generate_student_id(length=6):
"""生成指定长度的随机学号(整数)"""
return random.randint(100000, 999999) 6位学号范围
示例
student_id = generate_student_id()
print(f"随机学号:{student_id}")
```
二、生成随机成绩
成绩通常为0到100之间的数值,可生成整数或浮点数。
生成整数成绩
使用`random.randint(0, 100)`生成0到100的整数成绩。
```python
def generate_score(num_students=10):
"""生成指定数量的学生成绩(整数)"""
return [random.randint(0, 100) for _ in range(num_students)]
```
生成浮点数成绩
使用`random.uniform(0, 100)`生成0到100的浮点数成绩。
```python
def generate_float_score(num_students=10):
"""生成指定数量的学生成绩(浮点数)"""
return [random.uniform(0, 100) for _ in range(num_students)]
```
三、数据存储与统计
将学号与成绩关联存储,并进行统计分析。
使用字典存储数据
以学号为键,成绩为值,便于后续检索和统计。
```python
def store_scores(student_ids, scores):
"""将学号与成绩存储到字典中"""
score_dict = dict(zip(student_ids, scores))
return score_dict
示例
student_ids = [generate_student_id() for _ in range(20)]
scores = generate_score(20)
score_dict = store_scores(student_ids, scores)
```
按成绩排序
使用`sorted`函数按成绩升序排序。
```python
def sort_scores(score_dict):
"""按成绩升序排序字典"""
return dict(sorted(score_dict.items(), key=lambda x: x))
```
统计各分数段人数
使用`collections.defaultdict`统计各区间人数。
```python
from collections import defaultdict
def count_score_ranges(score_dict):
"""统计各分数段人数"""
ranges = [0, 60, 70, 80, 90, 100]
count = defaultdict(int)
for score in score_dict.values():
count[min(range, score)] += 1
return dict(zip(ranges, count))
```
四、输出结果
将统计结果以表格形式输出。
```python
def print_score_ranges(score_dict):
"""打印各分数段人数"""
ranges = [0, 60, 70, 80, 90, 100]
count = count_score_ranges(score_dict)
print("分数段统计:")
for r in ranges:
print(f"[{r}, {r+60}):{count[r]}人")
打印具体学生名单(按成绩降序)
sorted_scores = sort_scores(score_dict)
print("n学号t成绩")
for student_id, score in sorted_scores.items():
print(f"{student_id}t{score:.2f}")
```
完整示例代码
将上述步骤整合为一个完整脚本:
```python
import random
from collections import defaultdict
def generate_student_id(length=6):
return random.randint(100000, 999999)
def generate_score(num_students=10):
return [random.randint(0, 100) for _ in range(num_students)]
def store_scores(student_ids, scores):
return dict(zip(student_ids, scores))
def sort_scores(score_dict):
return dict(sorted(score_dict.items(), key=lambda x: x))
def count_score_ranges(score_dict):
ranges = [0, 60, 70, 80, 90, 100]
count = defaultdict(int)
for score in score_dict.values():
count[min(range, score)] += 1
return dict(zip(ranges, count))
def print_score_ranges(score_dict):